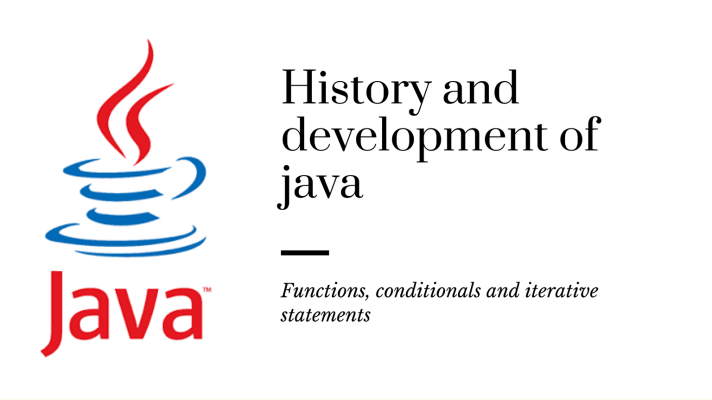
Learning Java is a basic today just like learning English. If you know English you can travel anywhere in the world. Similarly, if you know Java you can step into any aspect of programming and problem-solving. Java has wide usage in android and web apps development and much more. In our last few articles, we managed to bring you the basics of Java programming. So now let's dive deeper and know more about Java. In this article, we are going to read about functions. Functions are the basic building blocks of a program, we can never execute a program without having any function. This article is the third instalment of the ongoing java series. In this article, we are going to discuss library functions and some of the built-in function which is provided by the BlueJ. Apart from that the conditionals and iterative statements are also going to be included. All the program snippets are done in BlueJ code editor for Java. So now let's jump into the article and know what exactly are functions, conditionals and loops.
Built-in functions
Built-in functions are those which are pre-installed and pre-defined in the platform which we are using for performing a programming language.
Packages
Packages are the group of classes that performs a various function in a program. For the execution of a package, we have to include a package in our program by using the keyword import. Some of the packages are already installed when we write a program. The Math library is previously present in the program.
Some of the Math function are listed below:-
Math.max(): function can be used to take out the greatest number between the two.
int a= Math.max(6,9);
9
Math.min(): This function can be used to take out the smallest number between the two.
int a=Math.min(6,9);
6
Math.pow(): This function can be used to take out the raised power of the number.
int a=Math.pow(2,3);
8
Math.abs(): This function is used to take out the absolute value of a given number.
int a=Math.abs(-5.673);
5.673
Math.sqrt(): This function returns the square root of the given number.
int a=Math.sqrt(36);
6
Math.log(): This function returns the natural logarithmic value of a given number.
int a=Math.log(4);
0.602059991
Math.rint(): This function returns the exact value if the difference is less than 0.5 or else it returns the higher value.
double a=Math.rint(7.34);
7.0
double a=Math.rint(7.68);
8.0
Math.round(): This function returns the rounded off value.
double a=Math.round(6.25);
6.0
double a=Math.round(-7.89);
-8.0
Math.random(): This function returns a random value between 0 to 1.
double a=Math.random();
0.2538751474867425368
Math.ceil(): This function returns themes higher value corresponding to the argument given.
double a=Math.ceil(7.69);
8.0
Math.floor(): This function returns the lowest nearer small integer corresponding to the given value.
double a=Math.floor(7.98);
7.0
User-defined functions
In Java, it is possible to declare functions as per the user's requirement. The built-in functions and user-defined functions basically obey the same rules however the user has to define them as per its requirement in the whole program. As we know a function or method is a block of code which contains the mechanism of what is to be performed when called somewhere in the program. Some basic rules of user-defined function are:
- A function name is an identifier i.e only letters, numbers and a special character '_' is only allowed.
- A function name must not be a reserved keyword.
- A function name must not exceed 128 characters.
- A function name cannot start with numbers however numbers can be included in the middle or at the end.
- No spaces or special characters are allowed however in case of space, '_' can be used.
Java follows every aspect of object-oriented programming. Every function must be included inside a class i.e they should be a part of the class. A function is declared by its name followed by parenthesis '()'. At the time of declaring a method, the programmer has to declare what type of value does the function returns. In Java, return types can be
int, float, double, char, String, Map, List etc are allowed. If the function doesn't return any value, then its default return type is
void.
The programmer can use modifiers to define the access types whether it is public, private etc. The keyword static is used to define that the function can be accessed without creating objects. And at last inside the parenthesis, parameters are passed by declaring their types. A parameter is a value passed to a function.
public static int sum(int arg1, int arg2){
return arg1+arg2;
}
In the above snippet, two arguments are passed and the function will return their sum when called.
System.out.println(sum(3,5));
output: 8
Conditional Statements(if-else)
The statements which can be used in decision making are called Conditional Statements. In a conditional statement, we use the if-else statements.
if statement
We use if statement, when we have to check for a specific condition the if statement performs a specific task when the condition provided, is true or else it ignores the condition.
Syntax
if(condition)
{
Statement 1
Statement 2
}
For example, we write a code snippet to check whether you are a citizen and can vote or not :
If the given condition provided in the above syntax will be true then Statement 1 and Statement 2 will be executed and if not then control passes to the next code written in the program and skips it.
The only if statement
When there is a group of tasks and a particular task has to be done, in this situation we use the only if statement. In this type of if statement we can use multiple if and can give multiple conditions, provided if the condition is true then the statement corresponding to the condition will be executed and the control will come out.
Syntax
if (condition 1)
Statement 1
if (condition 2)
Statement2
if (condition 3)
Statement 3
For example, you need to check a number to be a single-digit, double-digit or triple-digit number.
If else statement
Here if the condition satisfies then the statements present inside the if block will be executed and if not then it will automatically print the statement written in the else block.
Syntax
if (condition)
{
Statement 1
Statement 2
}
else
{
Statement 3
Statement 4
}
For example, you've to check whether a number is even or odd :
Nested if statement
An if within an if is called a nested if statement. When there is two or more condition present after the execution of host conditions in this state we use nested if.
Syntax
if (condition 1)
{
if (condition 2)
Statement 1
else
Statement 2
}
else
{
if (condition 3)
Statement 3
else
Statement 4
}
For example, you've to check the greatest number among the three :
The Switch Statement
We have just seen that whenever that is a condition or more than one possibility is present we use the if statement, but in many cases the if statement proves to be very much complicated.
For example, the nested if is much more complicated when its branches go deeper. That's why it is a bit difficult to recognise if statement. To solve this problem Java provides with one more built-in function which is the Switch Statement.
Java provides us with multiple branching selection statements which are known as the Switch Statement. This function successively compares the value with the Case, whenever the case is matched with the value, the following block of statements associated with the Case gets executed.
Syntax
switch (expression)
{
case value 1:
statement 1;
break;
case value 2:
statement 2;
break;
case value 3:
statement 3;
break;
default :
default block;
}
For example, you've to do a menu-driven program to execute addition, subtraction, multiplication and division :
The statement which we right under the case must be terminated with a break. If we don't apply a break, the program will think that it has to proceed forward and it will execute the following cases also, that is why we use a break. When we use this break statement the control of the program come out from the Switch Statement.
When no match is found the program automatically execute the Default case.
Iterative statements
In programming, certain situations occur when the following task has to be repeated repeatedly. In those conditions we use Loops.
Loops are basically the functions which repeat the following task for a certain period. Basically, there are three types of loops used in Java :
- for loop
- while loop
- do-while loop
Constructions in a loop
- Variable initialisation: Before iteration, a fixed variable must be initialised so that it can control the loop.
- Test Conditions: The checkpoint where it is decided whether the loop will run or not. The Test Conditions may be present at the beginning of the loop or at the end of the loop. They are Entry control loop and exit control loop.
- Update expression: the update expression increases or decreases or we can say updates the value of the initialised variable. Basically, update expression is used for the next execution inside the loop.
- The frame of the loop: this contains the statement which is to be executed repeatedly as long as the test condition is true.
The For Loop
The most widely and frequently used loop in Java is the for loop, it is easy to understand and the syntax of this loop is much easier than the while and do-while loop. This is an entry control loop.
Syntax
for (initialisation; test condition; update expression)
{
The frame of the loop;
}
For example, you've to print the numbers 1 to 20 using for loop:
The While Loop
The second type of loop in Java is the while loop it is basically similar like the for loop but with little difference. The While loop is an entry control loop as well.
Syntax
while (condition)
{
The frame of the loop;
}
For example, you've to print the numbers 1 to 20 using while loop:
The following snippet iterate till the condition is true whenever it becomes false the control terminate out from the loop body.
The DO-WHILE Loop
The do-while loop is a bit different from the previously learnt for and while loop it is an exit control loop i.e. it executes the body first at once and after that checks the condition, if the condition proves to be true then it goes for the next iteration otherwise it terminate out of the loop.
Syntax
do
{
statement 1;
statement 2;
}while (condition);
For example you've to print the multiples of 2 :
The break statement
The break statement is one of the most important and most widely used statements in the Java programming language. Basically, the break statement is used to immediately terminate out from the following function. This is important because, if we don't use this then the control execute all the statement preceding it.
The continue statement
The Continue Statement is exceptionally used in looping. It is used whenever the programs need to iterate in the successive number (updations of control variable) without executing the previous code.
The return statement
The Return Statement is just like the break statement. Basically, it returns a value and after that, it exits out from the function. The basic function which makes it different from the Break statement is that it returns a value to the place where it was invoked.
Hope you enjoyed reading this Java tutorial article. In this article, the basics of java programming have been discussed. In the next article, we are bringing more exciting stuff. So, subscribe to our newsletter to stay tuned to our latest posts.
if you like the articles and want to read more such articles then subscribe to our newsletter to get the latest updates.
Share this article if you like and follow us on Facebook.
you're the true genius
ReplyDelete